Creating Dynamic Select Options in FilamentPHP: A Step-by-Step Guide
In FilamentPHP, dynamically populating select options can enhance user experience and streamline data entry.
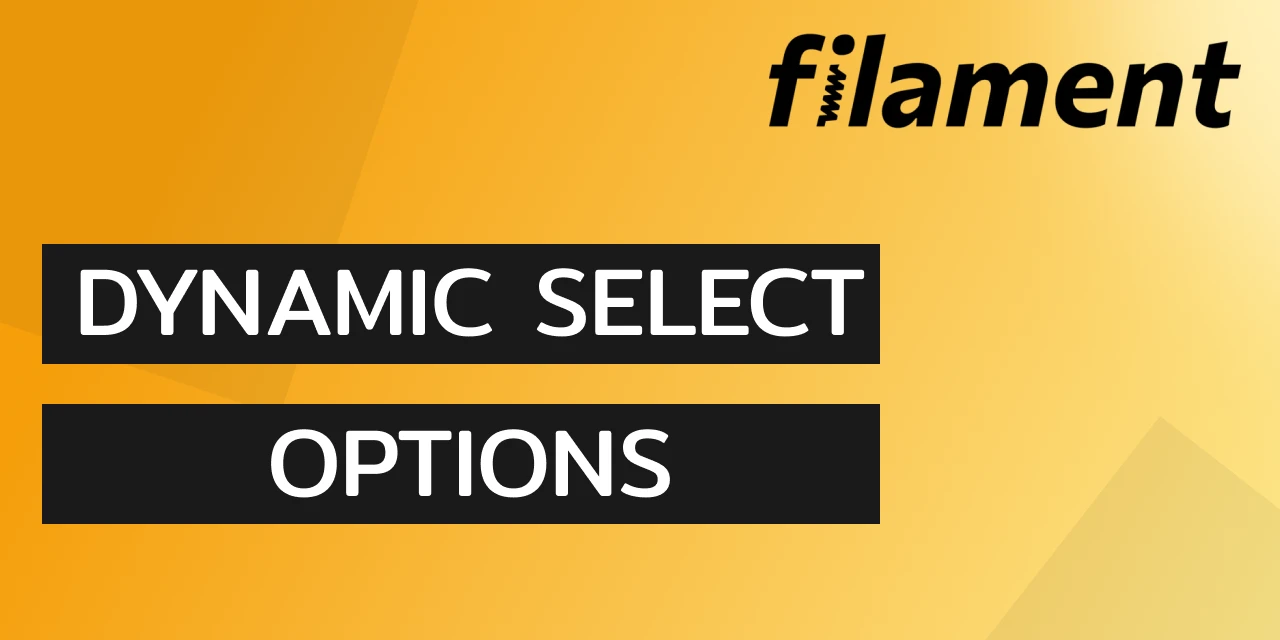
April 27, 2024
In FilamentPHP, dynamically populating select options can enhance user experience and streamline data entry. By fetching options from a database or an API, you can ensure that your select elements always reflect the most up-to-date information. In this tutorial, we'll walk through the process of creating dynamic select options in FilamentPHP.
Step 1: Adding `live()` and `searchable()` to the Select Component
In FilamentPHP, the `live()` method is used to make the select options dynamic, meaning the new value is reflected before hitting the submit button. The `searchable()` method adds a search bar to the select element, allowing users to easily filter through a large number of options.
use Filament\Forms\Components\Select;
Select::make('Country')
->live()
->searchable(),
Step 2: The `getSearchResultsUsing()` Method
The `getSearchResultsUsing()` method is used to define a callback that retrieves search results based on the user's input in the search bar.
Select::make('Country')
->live()
->searchable()
->getSearchResultsUsing(fn (string $search) => Category::query()
->where('name', 'like', "%$search%")
->take(5)
->pluck('name', 'id')
->toArray()
),
Step 3: Getting the Label of the Selected Option
The `getOptionLabelUsing()` method is used to define a callback that retrieves the label for each select option. This allows you to customize how the options are displayed in the select element.
Select::make('Country')
->live()
->searchable()
->getSearchResultsUsing(fn (string $search) => Category::query()
->where('name', 'like', "%$search%")
->take(5)
->pluck('name', 'id')
->toArray()
)
->getOptionLabelUsing(fn ($value): ?string => Category::find($value)?->name),
Select::make('Country')
->live()
->searchable()
->getSearchResultsUsing(fn (string $search) => Category::query()
->where('name', 'like', "%$search%")
->take(5)
->pluck('name', 'id')
->toArray()
)
->getOptionLabelUsing(fn ($value): ?string => Category::find($value)?->name)
->options([
'us' => 'United States',
'ca' => 'Canada',
'mx' => 'Mexico',
]),